Cleverly use GitHub Copilot to increase productivity - Part 1
It was only a matter of time before artificial intelligence technology’s power was to be harnessed towards processing programmable language. The logical reasoning of AI mixed with the immense pool of open source examples paved the way to the creation of the first stepping stone in an automated digital product creation environment.
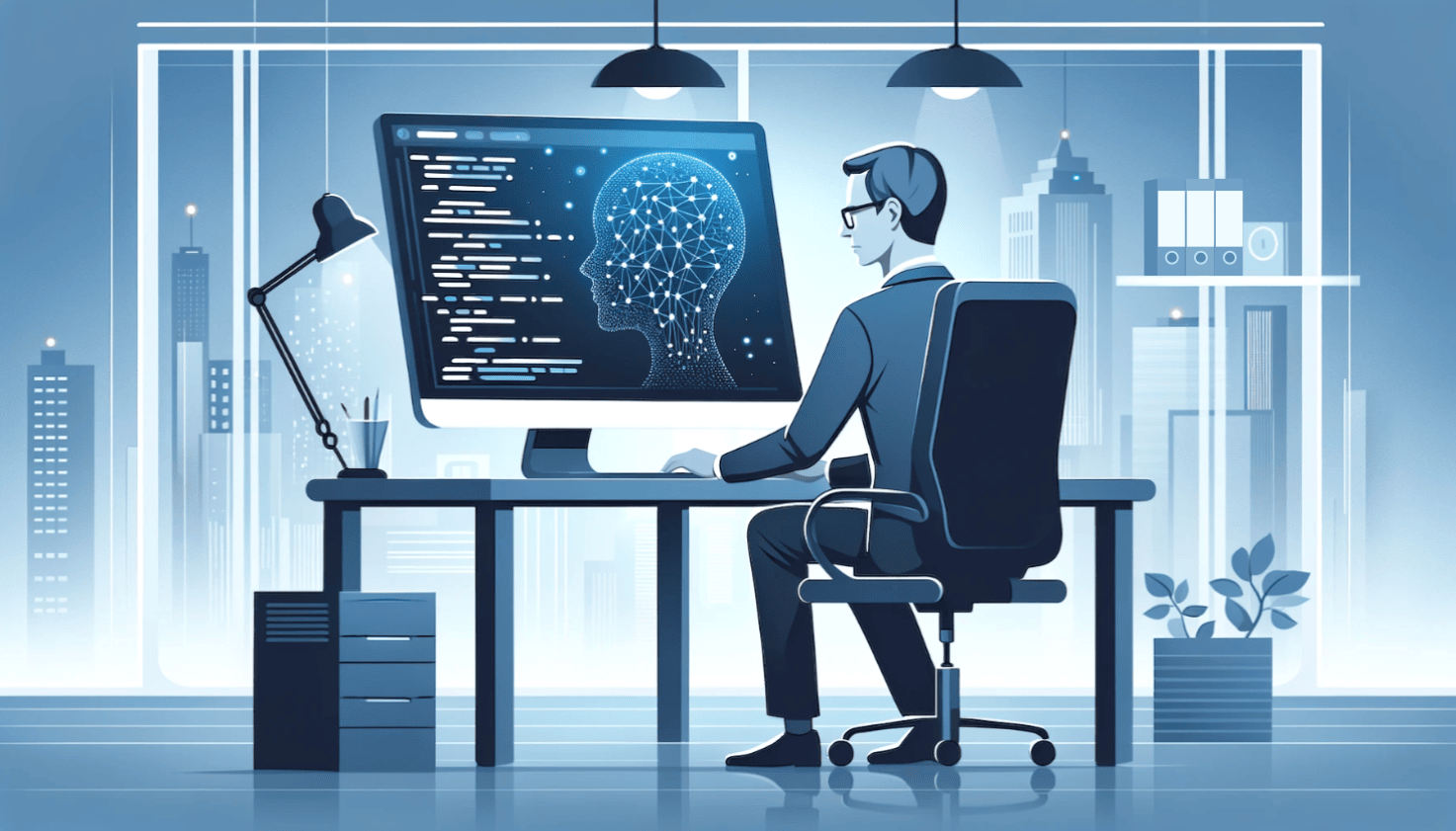
This article is the first part of a two part article about using Copilot to increase productivity. You can view the second part here.
In October 2021, OpenAI and GitHub released Copilot, a tool of the future aimed at helping developers increase productivity. This tool has been designed as a coding assistant lending a hand at any tasks related to building lines of code. It tries to predict in real time what's on your mind and what could be the next best path of action. Used properly, this tool can immensely increase developers productivity and it reduces trivial time-consuming code-related tasks such as writing trivial functions or adjusting existing code to new situations.
Like with any tool, one needs to be used wisely to achieve greatness. Otherwise, it will feel like any other gimmick on the market. Regarding Copilot, I've often seen developers buy a license, look at the autocomplete, find it funny the first few times then don't bother using it before continuing their work as usual. The key to harnessing the immense power of Copilot is to treat it like an "intern working for you". What it means is to give proper instructions on what has to be done and let it do its things before double checking what has been done as you don't want the code of an intern to be included in a product without proper reviewing.
Let's see the concept in action and look at the difference. Here is a simple example where a PHP function needs to be written about checking if a string is a palindrome (reads the same forwards and backwards). Since it is a pretty standard thing, the first reflex would be to write a function where a string is the input and a boolean is the output telling if it is a palindrome or not.
function isPalindrome($string) {
// Remove non-alphanumeric characters and convert to lowercase
$cleanedString = strtolower(preg_replace("/[^A-Za-z0-9]/", '', $string));
// Get the length of the cleaned string
$length = strlen($cleanedString);
// Check each character from the start and end, moving toward the center
for ($i = 0; $i < $length / 2; $i++) {
if ($cleanedString[$i] != $cleanedString[$length - 1 - $i]) {
// If any characters do not match, it's not a palindrome
return false;
}
}
// If all characters match, it is a palindrome
return true;
}
With copilot, you can ask to have a palindrome function be coded and tell how it should be done. The tool takes care of the rest.
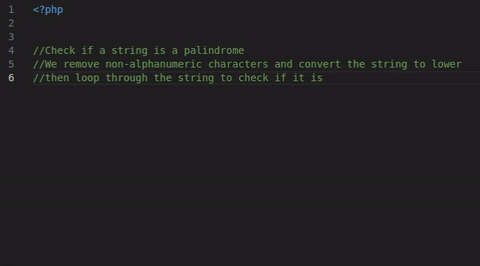
Impressive! Copilot gave us this code:
function isPalindrome($str) {
$str = strtolower(preg_replace('/[^a-zA-Z0-9]/', '', $str));
$len = strlen($str);
for ($i = 0; $i < $len / 2; $i++) {
if ($str[$i] != $str[$len - $i - 1]) {
return false;
}
}
return true;
}
By comparing both functions side by side, we can see that only variable names are different. The logic is exactly the same!
This is where it starts to get interesting. Depending on the speed of typing code without creating mistakes, it would have taken a certain amount of seconds/minutes to come up with the function manually. Instead, typing the comment and again based on your typing speed, would take less time to do. Plus, GitHub Copilot is also trained to write comments by explaining code. While you are typing an instruction, it can deduce what words are about to be typed next and auto complete the rest of the sentence thus further reducing the time it takes to achieve the end result. Extend this to an entire project, time saves are humongous and can greatly affect profitability.
One of the things to take into account is to not be greedy by trying to have the AI do too many things at the same time. It's crucial to cut the logic into small chunks of code that can be easily accomplished. It might mean writing more lines of code, but in the end, it will become more profitable for you, your team and the tool. More comments means more documentation which will make the code more understandable but also will give more information to the tool about the project in general, increasing the quality of the code it generates.
$mysql = new mysqli('localhost', 'root', '', 'test');
// Create a contact list with id, name, email of John Doe and Jane Doe as examples
$contacts = [
[
'id' => 1,
'name' => 'John Doe',
'email' => 'john.doe@email.com'
],
[
'id' => 2,
'name' => 'Jane Doe',
'email' => 'jane.doe@email.com'
]
];
// Loop every contact and create a custom key as an array
foreach ($contacts as $key => $contact) {
$contacts[$key]['custom'] = [];
}
// Contact the MySQL database through the $mysql variable and get
// the phone numbers into the custom array
$result = $mysql->query('SELECT * FROM contacts');
while ($row = $result->fetch_assoc()) {
foreach ($contacts as $key => $contact) {
if ($contact['id'] == $row['contact_id']) {
$contacts[$key]['custom'][] = $row['phone'];
}
}
}
Be wary of the generated code. Like an intern, it always needs to be reviewed by a human mind to prevent mistakes, vulnerabilities or any other harmful instructions having been inserted inadvertently. We must never forget that Copilot was trained on open source code found on the internet which might contain bad coding practices or malicious code. It should always be reviewed thoroughly.
Closing thoughts
GitHub and OpenAI released an incredibly powerful tool, giving smart developers a true edge in productivity. It removes the trivial and time consuming tasks of writing functionalities giving more time for programmers to design their code instead of trying to properly write it down into the current language. Every great thing has its drawback. It should be used with care and reviewed thoroughly making sure the quality is up with what could be done by a human mind.
Possibilities are endless but remember, creativity is the key.
Disclaimer: No AI models were used in the writing of this article. The text content was purely written by hand by its author. Human generated content still has its place in the world and must continue to live on. Only the image was generated using an AI model.